Swing
Swing是AWT的封装。
1. 窗口JFrame
public class Demo11 {
public static void main(String[] args) {
new MyJFrame2().init();
}
}
class MyJFrame2 extends JFrame {
public void init() {
JLabel jLabel = new JLabel("这是一个标签", SwingConstants.CENTER);
add(jLabel);
// 获得一个容器
Container container = getContentPane();
container.setBackground(Color.CYAN);
// 设置默认点击x的事件
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 200, 300);
setVisible(true);
}
}
2. 弹窗Dialog
public class Demo12 extends JFrame {
public Demo12() {
// JFrame 放东西 容器
Container contentPane = getContentPane();
contentPane.setLayout(null);
// 按钮
JButton jButton = new JButton("点击弹出一个对话框");
jButton.setBounds(30, 30, 200, 50);
// 点击这个按钮的时候弹出一个弹窗
// 这里用了lambda表达式,相当于
// jButton.addActionListener(
// new ActionListener() {
// @Override
// public void actionPerformed(ActionEvent e) {
// new MyDialog()
// }
// }
// );
jButton.addActionListener(e -> new MyDialog());
contentPane.add(jButton);
setBounds(100, 100, 700, 500);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new Demo12();
}
}
// 弹窗的窗口
class MyDialog extends JDialog {
public MyDialog() {
setVisible(true);
setBounds(100, 100, 500, 500);
Container contentPane = getContentPane();
contentPane.add(new JLabel("这是一个标签"));
}
}
3. 标签
label
new JLabel("xxx");
图标icon
public class Demo13 extends JFrame implements Icon {
private int width;
private int height;
// 无参构造
public Demo13(){
}
public Demo13(int width, int height){
this.width = width;
this.height = height;
}
public void init(){
Demo13 iconDemo = new Demo13(15, 15);
// 图标放在标签 也可以放在按钮上
JLabel jLabel = new JLabel("icontest", iconDemo, SwingConstants.CENTER);
Container contentPane = getContentPane();
contentPane.add(jLabel);
setVisible(true);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo13().init();
}
@Override
public void paintIcon(Component c, Graphics g, int x, int y) {
g.fillOval(x, y, width, height);
}
@Override
public int getIconWidth() {
return width;
}
@Override
public int getIconHeight() {
return height;
}
}
图片icon
public class ImageIconDemo extends JFrame {
public ImageIconDemo() {
JLabel jLabel = new JLabel("ImageIcon");
// 获取图片的地址
// 获取当前类下的资源
URL url = getClass().getResource("avatar.png");
assert url != null;
ImageIcon imageIcon = new ImageIcon(url);
jLabel.setIcon(imageIcon);
jLabel.setHorizontalAlignment(SwingConstants.CENTER);
Container contentPane = getContentPane();
contentPane.add(jLabel);
setVisible(true);
setBounds(100, 100, 200, 200);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new ImageIconDemo();
}
}
4. 面板
JPanel
public class Demo14 extends JFrame {
public Demo14() {
Container contentPane = getContentPane();
// 2行1列 垂直水平间距10
contentPane.setLayout(new GridLayout(2, 1, 10, 10));
JPanel jPanel1 = new JPanel(new GridLayout(1, 3));
JPanel jPanel2 = new JPanel(new GridLayout(1, 2));
JPanel jPanel3 = new JPanel(new GridLayout(2, 1));
JPanel jPanel4 = new JPanel(new GridLayout(3, 2));
jPanel1.add(new JButton("1"));
jPanel1.add(new JButton("1"));
jPanel1.add(new JButton("1"));
jPanel2.add(new JButton("2"));
jPanel2.add(new JButton("2"));
jPanel3.add(new JButton("3"));
jPanel3.add(new JButton("3"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
contentPane.add(jPanel1);
contentPane.add(jPanel2);
contentPane.add(jPanel3);
contentPane.add(jPanel4);
setVisible(true);
setBounds(100, 100, 400, 400);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo14();
}
}
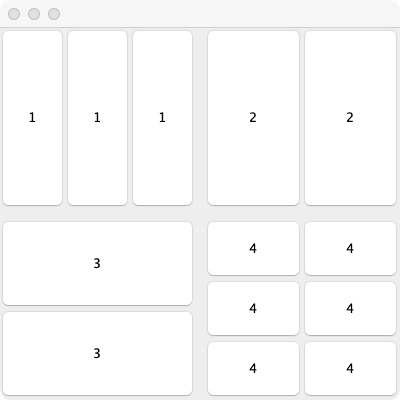
JScoll
public class Demo15 extends JFrame {
public Demo15() {
Container contentPane = getContentPane();
// 文本域
JTextArea jTextArea = new JTextArea(20, 50);
jTextArea.setText("这是一个文本域");
JScrollPane jScrollPane = new JScrollPane(jTextArea);
contentPane.add(jScrollPane);
setBounds(100, 100, 300, 350);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new Demo15();
}
}
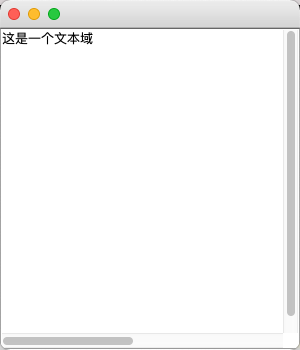
5. 按钮
图片按钮
public class Demo16 extends JFrame {
public Demo16() {
Container contentPane = getContentPane();
// 讲一个图片变为图标
URL resource = getClass().getResource("avatar.png");
ImageIcon imageIcon = new ImageIcon(resource);
// 把这个图标放在按钮上
JButton jButton = new JButton();
jButton.setIcon(imageIcon);
jButton.setToolTipText("把一个图片变成按钮");
contentPane.add(jButton);
setVisible(true);
setBounds(100, 100, 200, 200);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo16();
}
}
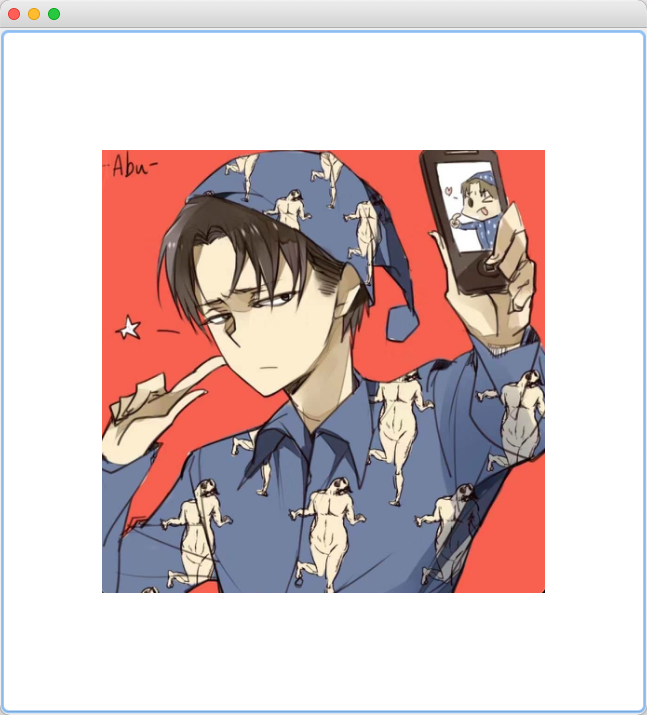
- 单选
public class Demo17 extends JFrame {
public Demo17() {
Container contentPane = getContentPane();
URL resource = getClass().getResource("avatar.png");
ImageIcon imageIcon = new ImageIcon(resource);
// 单选框
JRadioButton jRadioButton1 = new JRadioButton("JRadioButton1");
JRadioButton jRadioButton2 = new JRadioButton("jRadioButton2");
JRadioButton jRadioButton3 = new JRadioButton("jRadioButton3");
// 默认选中
jRadioButton1.setSelected(true);
// 由于单选框只能选择一个 分组 一个分组中只能选择一个
ButtonGroup buttonGroup = new ButtonGroup();
buttonGroup.add(jRadioButton1);
buttonGroup.add(jRadioButton2);
buttonGroup.add(jRadioButton3);
contentPane.add(jRadioButton1, BorderLayout.CENTER);
contentPane.add(jRadioButton2, BorderLayout.NORTH);
contentPane.add(jRadioButton3, BorderLayout.SOUTH);
setVisible(true);
setBounds(100, 100, 500, 500);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo17();
}
}
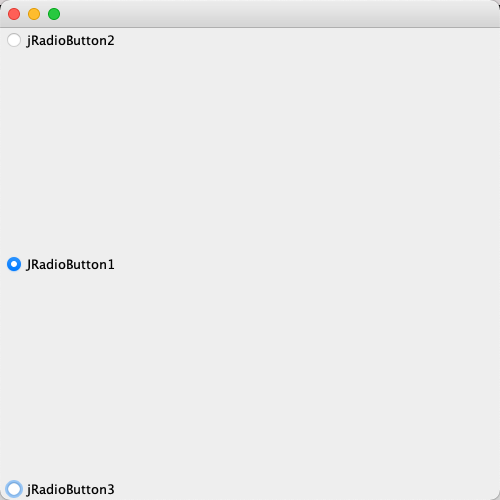
- 复选
public class Demo18 extends JFrame {
public Demo18() {
Container contentPane = getContentPane();
// 多选框
JCheckBox jCheckBox1 = new JCheckBox("jCheckBox1");
JCheckBox jCheckBox2 = new JCheckBox("jCheckBox2");
contentPane.add(jCheckBox1, BorderLayout.NORTH);
contentPane.add(jCheckBox2, BorderLayout.SOUTH);
setVisible(true);
setBounds(100, 100, 200, 200);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo18();
}
}
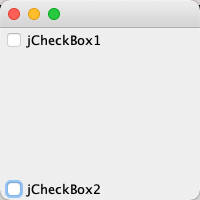
6. 列表
- 下拉框
public class Demo19 extends JFrame {
public Demo19() {
Container contentPane = getContentPane();
// 下拉列表框
JComboBox<Object> status = new JComboBox<>();
status.addItem("正在热映");
status.addItem("已下架");
status.addItem("即将上映");
contentPane.add(status);
setVisible(true);
setBounds(100, 100, 200, 200);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo19();
}
}
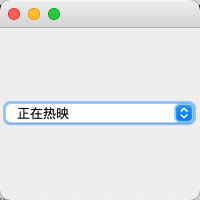
- 列表框
public class Demo20 extends JFrame {
public Demo20() {
Container contentPane = getContentPane();
// 生成列表的内容
// String[] contents = {"1", "2", "3"};
Vector<String> contents = new Vector<>();
JList<String> stringJList = new JList<>(contents);
contents.add("1");
contents.add("2");
contents.add("3");
contentPane.add(stringJList);
setVisible(true);
setBounds(100, 100, 200, 200);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo20();
}
}
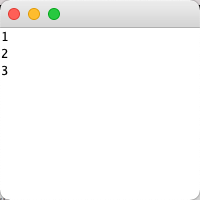
7. 文本框
- 文本框
- 密码框
- 文本域
public class Demo21 extends JFrame {
public Demo21() {
Container contentPane = getContentPane();
// 文本框
JTextField jTextField = new JTextField("hello");
JTextField jTextField2 = new JTextField("world");
contentPane.add(jTextField, BorderLayout.NORTH);
contentPane.add(jTextField2, BorderLayout.SOUTH);
// 密码框
JPasswordField jPasswordField = new JPasswordField("password");
jPasswordField.setEchoChar('*');
contentPane.add(jPasswordField, BorderLayout.CENTER);
// 文本域
JTextArea jTextArea = new JTextArea("这是一个文本域");
contentPane.add(jTextArea, BorderLayout.EAST);
setVisible(true);
setBounds(100, 100, 200, 200);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Demo21();
}
}
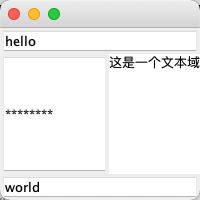